TOPIC A: Basics
Contents :
Cardinal, Integer, + - * DIV MOD, IF,
FOR, WHILE, RdCard, WrCard,
RdInt, WrInt, WrStr, WrLn.
Exercises :
D0: First Steps
1) Your first Modula 2 program:
- open Modula2 compiler XDS: start -> programs
-> [TW]
Programming ->
XDS environment
- create new file
- copy HelloWorld to an
empty sheet
- save as HelloWorld.mod => must
be
exactly this name, you must add .mod yourself
- the path to this file may not contain spaces anywhere
- run the program (if the blue running man
is grey instead
, open another sheet and return back
- this is probably a bug of the XDS environment)
2) A very simple example using
variables:
- open Modula2 compiler XDS
- create new file
- copy FirstVars to an
empty sheet
- save as FirstVars.mod
- run the program
3) A very simple example using user
input:
- open Modula2 compiler XDS
- create new file
- copy FirstInput to an
empty sheet
- save as FirstInput.mod
- run the program
D1: Divisible
open Modula2 comp
open Modula2 compiler XDS: start -> programs
-> [TW]
Programming ->
XDS environment
create new file
copy the solution D1Part1
save as D1Part1.mod (must be the exact same name!!
Don't
forget the .mod)
je creeert het best een folder per topic!
PAS OP: folders of programma's mogen geen spaties
bevatten
(dat geraakt XDS in de war)
- run het programma (blauw mannetje
)
- als je niet kan runnen (blauw mannetje is grijs): file terug
sluiten
en heropenen
- bekijk het programma en probeer het te begrijpen (lees hieronder
de
opgave)
- bekijk daarna deel 2 (solution
D1Part2) en deel 3 (solution
D1Part3)
- nu ben je klaar om zelf te programmeren...
- Ask the user for a number x and tell if it is a divisible by 7.
- Make sure that x is greater than 10000, otherwise give an error
message.
- Repeat this 5 times (ask + check). Also print the iteration
number.
- Don't repeat it 5 times, but repeat it until x is divisible by
7.
Also give the number of iterations.
- Ask in the beginning of the program the number by which x must
be
divisible (instead of the 7).
- Ask in the beginning of the program the number where x must be
greater
of (instead of the 10000).
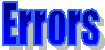
D2: Teken figuur
open de Modula2 compiler XDS: Start -> Programs
->
[TW] Programming ->
XDS environment
creer een nieuwe file
copieer de oplossing Demo2
save als Demo2.mod (Dit moet exact deze naam zijn!!
Vergeet
vooral de .mod niet!!)
save in je eigen drive (best de E:), maak er een
folder
voor deze oefeningen (bvb "Modula2" )
je creeert het best een folder per topic (bvb TopicA, TopicB,
...)!
PAS OP: folders of programma's mogen geen spaties
bevatten
(dat geraakt XDS in de war)
Zoals je ziet in de IMPORT sectie, heb je Wimdows nodig om het grafische
scherm te kunnen gebruiken. Download dit op onze technology pagina.
- run het programma (blauw mannetje
)
- als je niet kan runnen (blauw mannetje is grijs
): file terug sluiten en heropenen
- bekijk het programma en probeer het te begrijpen (lees hieronder
de
opgave)
- nu ben je klaar om zelf te programmeren...
Opgave: vraag een kleur aan de gebruiker ('R' voor rood,
'B'
voor blauw, 'G' voor groen, gebruik RdKey() om een
letter in
te lezen), teken dan verschillende geometrische vormen op het scherm
(cirkel,
lijn, rechthoek)
Bestudeer Demo2b, hier
worden 10
lijnen getekend mbv een FOR-statement
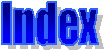
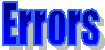
S1: Bla
- Print "bla" 10 times to the screen.
- Ask the user first how many times "bla" must be printed, then do
it.
Hint: copy the solution of Demo1 part3 and modify it
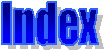
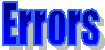
S2: Arrow
*
**
***
**
*
- Print the above arrow to the screen.
- Ask the user first how big the arrow must be (in our case the
arrow
is 3 wide).
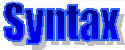
S3: Division & Modulo
- Make a program that asks for two numbers x & y, and
calculates
the division (deling) and the modulo (rest)without using
the
*, /, DIV or MOD operators (only + and -).
Hint: once your program runs, but is not gicing the right
results,
check your program with the debugger, as explained here.
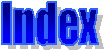
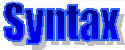
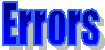
S4: Teken Lijnen
- Vraag de gebruiker om 2 punten in te geven (x1, y1, x2, y2 : dus
4
gehele getallen). Teken een lijn tussen deze beide punten.
- Controleer of de ingegeven punten wel degelijk binnen het
grafische
scherm liggen (0<x<GRAPH_WIDTH; 0<y<GRAPH_HEIGHT), anders
geef
je een foutmelding en teken je niets op het scherm!
Tip: D2 geeft een voorbeeld van hoe je het grafisch scherm kan
gebruiken. Vergeet niet Wimdows te downloaden op onze technology pagina om de
code te kunnen compileren!
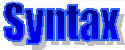
S5: Draw Grid
- Teken een grid (raster) op het scherm van 500x500, met een
interlinie (tussen de gridlijnen) van 50 pixels.
- Vraag de gebruiker voor de interlinie en teken dan de grid.
- Vraag de gebruiker voor het aantal gridlijnen en teken de
grid.
In english:
- Draw a grid on screen (a grid of lines)
- Ask user for spacing in between gridlines, draw grid (FOR TO BY
END;)
- Ask user for amount of gridlines in X & Y direction, draw
grid
(DIV)
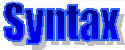
S6: Gestreepte Ruit
- Teken een ruit in het midden van het scherm (zo groot mogelijk,
tegen de randen).
- teken N vertikale lijnen in de ruit op gelijke afstand van
elkaar.
N is een gegeven constante die aanpasbaar moet zijn. Dan bekom je dit:
N = 5
N
= 4
Hint:
- bereken op papier de formules voor de eindpunten van de lijnen.
- als uw programma werkt maar de lijnen niet juist tekent, kan je
je
fout(en) met de debugger zoeken!
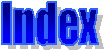
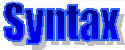
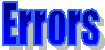
X1: Sequence
The following formula defines a sequence of cardinals:
N<1> = 1 (first, initial element)
N<i> = (N<i-1> * 5 + 356) MOD 97 (next elements)
with i: 1,2,3,4,...
so: N<2> = (N<1> * 5 + 356) MOD 97 = (1 * 5 + 356) MOD
97
= 70
- Print the series for i up to 20.
- Print the series until it is 1 (the initial value) again. Print
the
number of elements you get (without counting the last 1 again).
- Calculate and print the average value of the series (without
counting
the last 1 again).
- Question: What is the maximal value N<i> can have, regardless
of
the initial value. Divide this number by 2.
- This formula can be used to generate random numbers between 0 and 96.
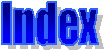
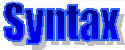
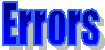
H1: Multiples ("Veelvouden")
- Ask the user for two numbers x & n. Print the n multiples of
x:
- eg x=24
- "Multiple 2 of 24 = 48"
- "Multiple 3 of 24 = 72"
- ...
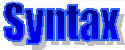
H2: Fibonacci
The following formula denote the Fibonacci series:
N<i> = N<i-1> + N<i-2> for
i
> 1
with N<0> = 1, N<1> = 1
This results in: N<2> = 2, N<3> = 3, N<4> = 5, ...
- Write a program that calculates and prints the first n fibonacci
numbers,
with n given by the user.
- Replace the FOR by a WHILE.
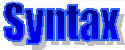
T1: Output
- What is the output of the following program? Find it out on
paper.
MODULE T1;
<* NOOPTIMIZE + *> (* this is for using the debugger *)
FROM IO IMPORT WrLn, WrCard, RdCard;
VAR x1, x2, x3: CARDINAL;
BEGIN
x1 := 3;
x2 := RdCard(); (* hier tik ik 7 in (here
I
type 7) !! *)
WHILE (x2 < 30) DO
x3 := x1 + x2;
IF (x3 MOD 3) = 0 THEN
x2
:= x2 + x3;
ELSE
x2
:= x2 - 1;
END;
END;
WrCard(x1, 0);
WrLn();
WrCard(x2, 0);
WrLn();
WrCard(x3, 0);
WrLn();
END T1.
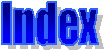
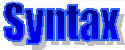
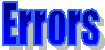
T2: Debug
A student wrote the following program for exercise S2
(Arrow).
It runs, but there are 2 errors!! Find and correct them.
See
for tips.
MODULE T2;
<* NOOPTIMIZE + *> (* this is for using the debugger *)
FROM IO IMPORT WrStr, WrLn, RdCard,
WrCard, RdInt, WrInt;
VAR n, i,
j:CARDINAL;
(*
variable-declarations *)
BEGIN
WrLn;
n := 5;
FOR i := 1 TO n DO
REPEAT
j := j + 1;
WrStr("*");
UNTIL (j = i);
WrLn;
END;
FOR i := n-1 TO 1 BY -1 DO
REPEAT
j := j + 1;
WrStr("*");
UNTIL (j = i);
WrLn;
END;
END T2.
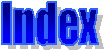
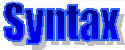
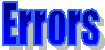
T3: Output
What is the output of the following program? Find it out on
paper.
MODULE T3;
<* NOOPTIMIZE + *> (* this is for using the debugger *)
FROM IO IMPORT WrLn, WrCard, RdCard;
VAR i, j: CARDINAL;
BEGIN
FOR i := 10 TO 22 BY 5 DO
j := i;
REPEAT
j := j - 2;
UNTIL (j MOD 3) = 0;
WrCard(j, 0);
WrLn();
END;
END T3.
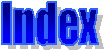
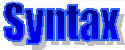
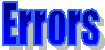